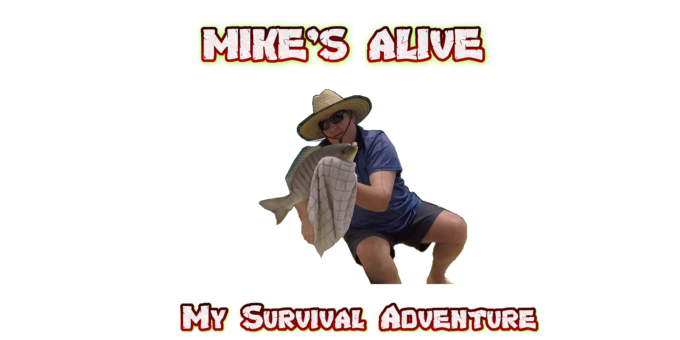
Hi everyone. Today I’m going to show you how to create a basic html web page and run it on your pc or laptop even if you aren’t connected to the internet.
This is the first tutorial in a series where I will show you step by step how to create an awesome browser game using html, css and javascript, with maybe a bit of php thrown in for extra goodness. I hope you try this at home and have fun learning along with me.
This is a very handy way of practising your coding if you don’t have web hosting or a domain name.
This method works for windows, apple and linux systems. I will be using Linux Mint.
So let’s get started.
Install a Text Editor
First off you will need a text editor that has a “Live Server” function. For this I use and recommend Visual Studio Code also known as VSCode, but you could use any alternative.
Now, it is possible to write html pages using just your notepad but that is a different method that I may cover in a later video. For now, install a good text editor and we’ll move to the next step.
To the next step!!
Create Your Working Folder and HTML File
For the purposes of this tutorial, create a folder on your desktop and call it coding or practise or whatever you like.
Ok, now go ahead and open your text editor.
Click “create a new file”. You can name your file anything but for now let’s stick with a standard name for a basic html page such as “index.html”
Save the file to your working folder.
And let the fun begin!
The code for your html file needs to be laid out with a certain structure in order for your page to display properly in a web browser.
In order to do this I’m going to use a powerful feature built into VSCode and other good text editors to automatically generate a basic html file then we’ll go through the parts and see what does what. Later you can try writing the page from scratch but you’ll always have this method to fall back on and I’ll also provide a boilerplate template at the end of this tutorial.
But without further delay, let’s crack on with your html file.
How to Create an HTML Boilerplate
So with the blank file open in VSCode (or your alternative), type an exclamation mark and press “enter” on your keyboard.
Whammo! Like magic, your text editor creates a basic html page complete with everything it needs to display in a web browser. This basic page is known as a “boilerplate”, and is the foundation of all html web pages.
Hit ctrl-s or click the save icon in your text editor to save the generated page, and overwrite the blank version.
Now, in your text editor, go to the View settings and open the Explorer sidebar. Inside the Explorer, navigate to the desktop or the folder where you saved your index.html file.
Right-click on the index.html file name and select “Open with Live Server”. This will open the file as a web page in your default browser.
It’s alive!! Hooray!
Ok, at this stage all you have is a blank page but don’t stress – we’ll liven it up pretty quick. Let’s jump back into the code…
Take a look at the various elements of the html. You will use these on almost every web page you ever build so you will want to familiarise yourself with them.
The <!DOCTYPE html> tag at the top tells your browser that this is an html document. Pretty crazy, right?
Note that “DOCTYPE” is in capital letters whereas all other tags are written in lower-case. This is the convention although browsers will usually ignore the differences. We will stick with the convention for the purposes of this tutorial.
The opening <html> tag follows the DOCTYPE, and its counterpart, the closing </html> tag appears at the very bottom of the page. The rest of your code should be written between this pair of tags.
The pair of HTML tags look like this:
<html lang=”en”>
</html>
The lang declaration inside the opening html tag tells the browser the language used for your page. It is not strictly required but should be included on your production sites.
The forward slash indicates a “closing” tag. Most tags on an html page need to be closed in this way. There are a few exceptions though, which we will learn about later.
Now let’s take a look at the content between the opening and closing html tags.
The first section below the opening html tag is called the head. This is where a lot of the behind-the-scenes work is done. For example, in the head you can declare meta properties for your page; link to external resources; declare on-page styles; and control various other data. Most of the content of the head is not shown directly on the web page itself but everything has a job to do.
The <meta> tags help web browsers and search engines to display your web page and can also serve a large number of other functions.
In the boilerplate example, meta tags are used to tell web browsers which character set should be used to encode the html, and the initial viewport settings. More on these in a later tut.
The most useful part of the head for us at this stage is the <title> tag. The title tag sets the page name that is displayed in the tabs on web browsers. Note that by default the name of this page is declared and displayed as “Document”. Let’s try to change that now.
Click in between the quotes and change the page title to “My html page” then click ctrl-s or the “save file” icon in your editor.
Notice that the Live Server automatically updates the name of your page in the tab of your browser. If the name in the tab hasn’t changed, try refreshing the page inside the browser itself.
Alright. Now you can start writing the actual content that will appear on your web page.
You do this between the <body></body> tags. Place your cursor between the pair of body tags and hit enter a few times to make some space. Like this:
<body>
</body>
Now on the line just below the opening <body> tag, type “My web page” without the quotes, then hit ctrl-s or save.
Look at your browser now and you’ll see “My web page” written on the page. All good.
You could write anything in the body, but it will show up as plain text with no style other than the browser’s default setting. We can do better.
Wrap the text with a pair of <h1></h1> tags like so:
<h1>My web page</h1>
Save the file again and check your browser.
Your web page now has a nice big heading.
You could also make some smaller headings by using similar tags such as h2, h3, h4. These smaller headings are usually used at the top of sections and sub-sections of a page. Even smaller headings are recognised and displayed by most browsers but really, why would you bother?
Let’s just create an h2 below the h1 like so:
<h1>My web page</h1>
<h2>My section heading</h2>
And now add a paragraph which is where you would normally write your content such as a blog post, news article or what have you. Use the <p></p> tags for this, with your new text in between them.
<p>This is my new paragraph. Oh, what a story!</p>
Save the file and check the results in your browser.
Impressive… most impressive!!
If the paragraph hasn’t displayed properly, check your closing tags.
Ok, now that we have a couple of headings and a paragrph, let’s see if we can make them look more appealing. They need some style!
Style refers to the appearance of the page itself including the background, your text, and other elements that you might want to add.
Go up to the head section in your html file. Just below the title declaration enter the following to increase the size of the text on the page.
<style>body{font-size:60px;}</style>
So here we have opened a <style> tag then used a selector of “body” which means browsers will display the body of your page using these style settings. We have named an attribute “font-size” and given it a value of 60px (pixels). The new size will be applied across the board, and will automatically be adjusted for headings so h1 headings will still be larger than h2, h3, etc.
Save the file now and check the new look in your browser. Larger text is always easier to read.
Sometimes you might only want to change the size of text within your paragraphs. In this case you would change the selector from “body” to simply “p”. This will give your paragraphs the large size but your headings will be at the default. Let’s see how that would work…
<style>p{font-size:36px;}</style>
Check the result as before and you will see the rule is only applied to the paragraph. If you created a second paragraph using another pair of <p></p> tags it would also be displayed using this rule.
We can restore the body rule as before which will make the headings much larger than the paragraph text. Just leave a space between each new rule as you go. Try this:
<style>body{font-size: 60px;} p{font-size:36px;}</style>
Rules applied to the body are overridden by later rules that target a particular element type. This can be confusing when you’re building large sites so we tend to create special “classes” and then add those classes to elements in the html page as desired. That is fun for another day.
Now all your text is larger but paragraph text is slightly smaller by comparison. You can make your headings really big or small according to your needs by creating a separate rule for h1, h2, etc.
A Special Note About h1 Headings
By convention a web page should only ever have one h1 heading, and this is usually reserved for the page title. Use your h2, h3, h4 etc as you move to less important headings.
Ok, we’re going to add a touch of color to our page to make it jump off the page and grab us by the eyeballs.
You may be familiar with html color codes. These codes tell browsers what color your text should be. In html you can often just write the name of a common color such as black, blue, red, etc, or define it using a hex code such as #000 for black or #fff for white, or by assigning an rgb value like rgb(59, 59, 59).
Try this example:
Replace the <style> line with the following to change the body background to black and all text to white:
<style>body{background-color:black;color:white;}</style>
The darker background can make reading web pages easier on the eye. But you can also change the color of each element type as well. Let’s change the color of only the h1 heading to blue while leaving the rest white.
<style>body{background-color:black;color:white;} h1{color:blue;}</style>
Let’s put a few of these together now to make our web page really pop!
<style>body{background-color:black;color:white;font-size: 48px;} h1{color:blue;} h2{font-weight:500; font-style: italic;} p{color: chartreuse;}</style>
There are many style parameters that can be changed in this way. Theoretically these changes can all be done in the head section but generally we would move the content of the <style></style> tags to a separate file known as a cascading style sheet and create a link to it using the <link> tag inside the head.
Let’s go ahead and do that now.
In your text editor click “New File” and name it style.css. Save it inside your coding folder next to the index.html file.
Alright, now we’re going to cut and paste everything between the <style></style> tags from the index.html and paste it straight into the empty css file we made.
Grab this line:
<style>body{background-color:black;color:white;font-size: 48px;} h1{color:blue;} h2{font-weight:500; font-style: italic;} p{color: chartreuse;}</style>
And paste it at the top of your style.css file. Delete the <style></style> tags from the line, then save the file.
Now in the part of your head section where you cut that line from, enter the following line to create a link to the css file.
<link rel=”stylesheet” src=”main.css”>
Now whenever you load your html file your browser will know where to grab the styles from. Using a separate css file in this way makes it much easier to change the appearance of your webpage on the fly, and is very important on sites with a lot of pages.
It is normal practice to break css rules apart so they are easier to read and amend. Do this by inserting a line after each “rule” (or set of curly brackets).
Your style.css file should now look like this:
body{background-color:black;color:white;font-size: 48px;}
h1{color:blue;}
h2{font-weight:500; font-style: italic;}
p{color: chartreuse;}
Try changing a couple of settings in your css file and see how they are applied to your web page. Remember to save the style.css file after making each change, and refresh the browser if necessary to pull the changes onto the page.
Awesome.
So a quick recap of what we’ve done so far.
First, we installed a legit text editor such as VSCode or similar to give us more power than a simple notepad.
We then created a folder to keep our code/files in.
We went ahead and used the text editor to create an html boilerplate.
Next we created a couple of headings and a paragraph using the appropriate tags: <h1></h1>, <h2></h2>, <p></p>.
After that we created some nice styles for these elements and the page body using style tags within the head section.
And finally we created an external stylesheet to hold all these css rules, and used the <link> tag in our head to call it when our index.html file is run in a browser.
And this is our code as it stands:
First, the index.html…
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Document</title>
<link rel=”stylesheet” href=”style.css”>
</head>
<body>
<h1>My web page</h1>
<h2>My section heading</h2>
<p>This is my new paragraph. Oh, what a story! This is my new paragraph. Oh, what a story! This is my new paragraph. Oh, what a story! This is my new paragraph. Oh, what a story!</p>
</body>
</html>
And here is the style.css…
body{background-color:black;color:white;font-size: 48px;}
h1{color:blue;}
h2{font-weight:500; font-style: italic;}
p{color: chartreuse;}
That is a quick how-to for creating a basic html web page using html and css.
The next step would be to add more text and some images, then use divs, classes and id’s to group and style various elements in different ways. I will cover these in more tutorials.
I hope you have enjoyed this introductory how-to and will go on to learn more and build some amazing web pages.
See you next time!